(Updated 8/12/23)
According to the Expo docs, you cannot run Firebase Analytics alongside Expo Go. I think this is misleading, because in my experience, you can get Analytics running, you just can’t call it from within Expo Go. You can call Analytics from apps built with eas
, which is likely what you are running in production anyway.
Here are the steps I took to set up Firebase Analytics for iOS. I am not sure if this will work for Android builds.
Installing packages
You will need to install several packages from your command line.
> npx expo install expo-dev-client
> npx expo install expo-build-properties
> npx expo install @react-native-firebase/app @react-native-firebase/analytics @react-native-firebase/perf @react-native-firebase/crashlytics
Create your Firebase project
Add a new project from the Firebase console: https://console.firebase.google.com/u/0/
Enter your project name
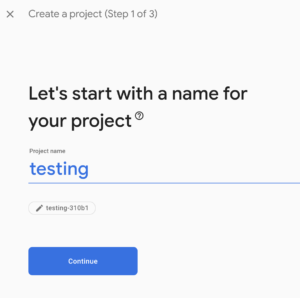
Make sure you select “Enable Google Analytics for this project”. You may have to go through additional setup if you haven’t previously used Google Analytics.
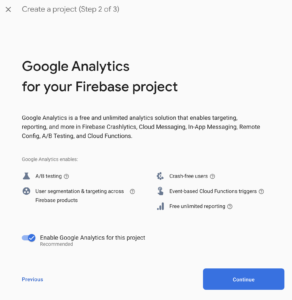
Select the Analytics account, and press Create Project
:
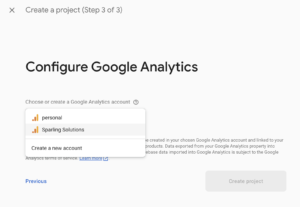
Configure your Firebase project
Once the project is created, select it from the dashboard if you aren’t already on the project page.
Now, select the iOS
option from “Get started by adding Firebase to your app” page.
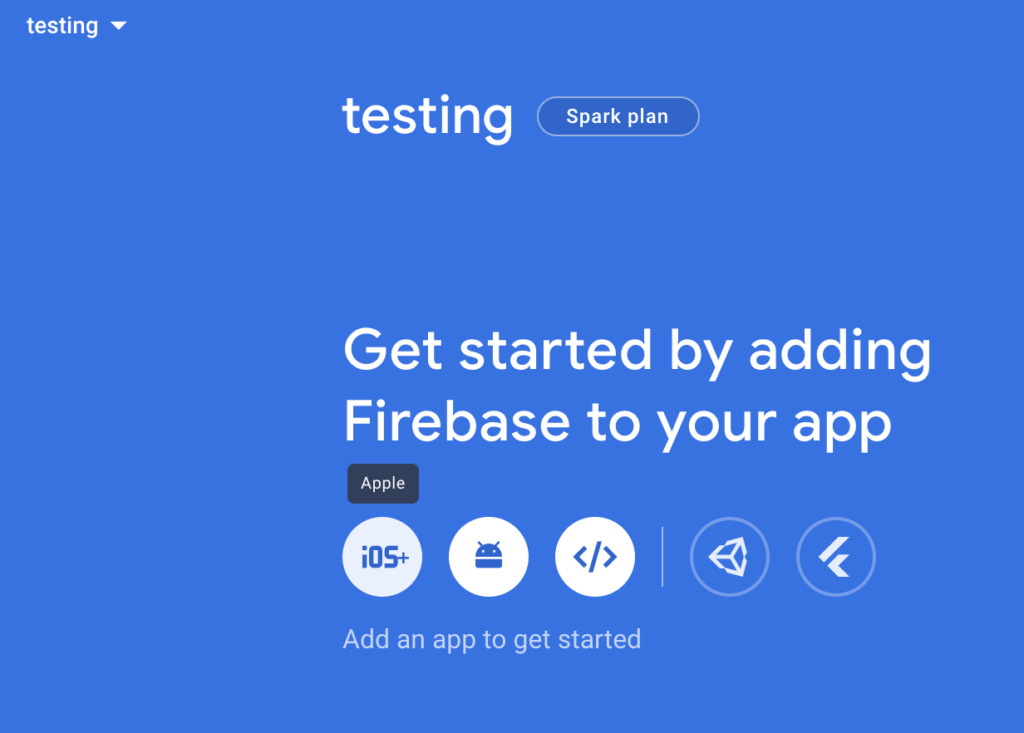
There are several steps on the page, but you only need to do the first couple.
Register your app
You can find your Bundle ID in the bundleIdentifier
field of the app.json
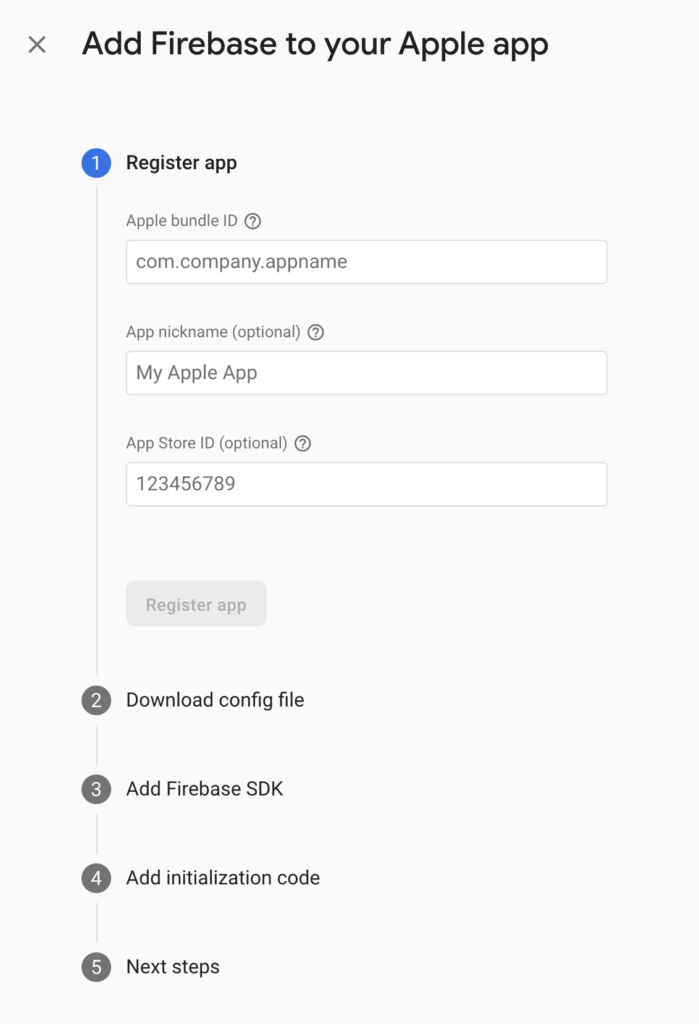
Download the GoogleService-Info.plist
file
Put the file in the root directory of your project. This should probably not be checked into Git, but I don’t think it is too critical.
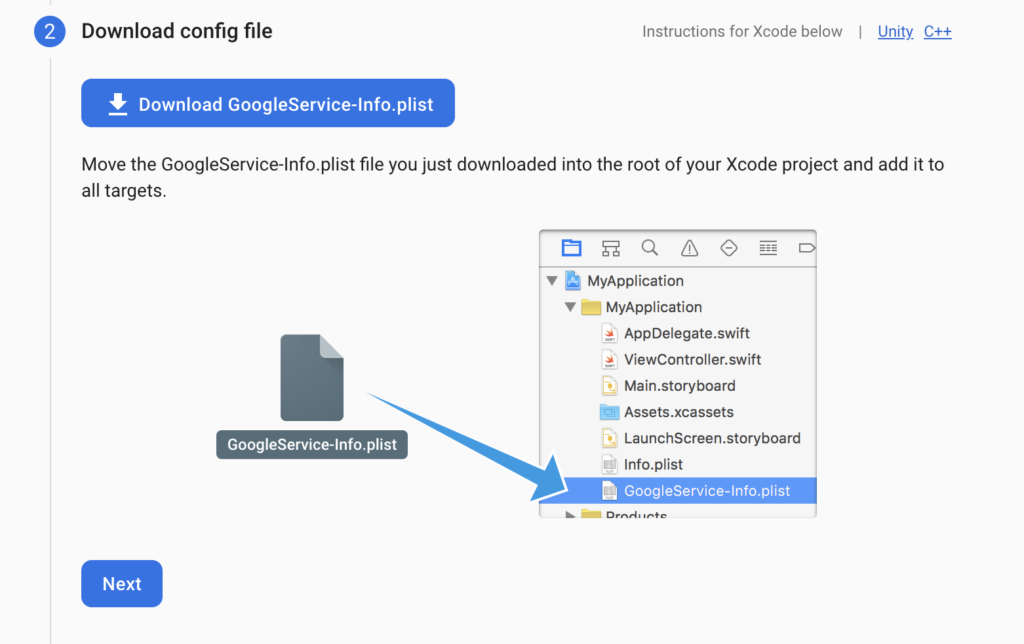
You can skip the rest of the steps on the page (SDK, initialization)
App Configuration
You need to add a couple of items to your app.json
file.
"ios": {
<...other setup if present>
"googleServicesFile": "./GoogleService-Info.plist"
},
"plugins": [
<...other setup if present>
"@react-native-firebase/app",
"@react-native-firebase/perf",
"@react-native-firebase/crashlytics",
[
"expo-build-properties",
{
"ios": {
"useFrameworks": "static"
}
}
]
],
Calling Firebase from React Native code
Expo Go cannot load @react-native-firebase/analytics
, so create a wrapper file that will conditionally load it if we aren’t running the app in Expo Go. The code below includes a function logLevelComplete
, that takes two parameters: level
and moves
, and logs a custom event called level_complete
.
import Constants, { ExecutionEnvironment } from 'expo-constants'
// `true` when running in Expo Go.
const isExpoGo = Constants.executionEnvironment === ExecutionEnvironment.StoreClient
let analytics
if (!isExpoGo) {
// eslint-disable-next-line @typescript-eslint/no-var-requires
analytics = require('@react-native-firebase/analytics').default
}
export async function logLevelComplete(level: number, moves: number) {
if (isExpoGo) {
console.log(
'levelComplete analytics event, level: ',
level,
'moves: ',
moves
)
} else {
await analytics().logEvent('level_complete', { level: level, moves: moves })
}
}
Now, you can log events by calling the functions you create in firebaseWrapper.ts
. If you are running in Expo Go, it will log the event to the console, if you are running the app through an eas
build, it will send the Firebase events to Firebase.
Running in Expo Go with the simulator
Prior to Expo 49
You can run Expo Go as you normally would, but you will see a warning in your logs that indicates the build is not installed in your simulator, you can ignore this warning.
The expo-dev-client package is installed, but a development build is not installed on iPhone 12 mini.
Launching in Expo Go. If you want to use a development build, you need to create and install one first.
Learn more
Expo 49
In Expo 49, npx expo start
will default to development
builds rather than Expo Go if expo-dev-client
is installed.
To force Expo Go to be used, launch with: npx expo start --go --clear
If you don’t, you will get an error like the one below, and the app won’t launch. You can also switch to Go by pressing s
:
› Opening on iOS...
CommandError: No development build (<appname>) for this project is installed. Please make and install a development build on the device first.
Learn more
If you want to run the application natively, and not through Expo Go, I think you will need to build a preview version of the app through the eas
command. This takes a while to compile, and doesn’t work for my workflow. I believe these instructions will help you get that set up, but I haven’t tried it: https://docs.expo.dev/develop/development-builds/create-a-build/#create-a-build-for-emulatorsimulator
Running on your device
To test that the Firebase integration is working, create an eas preview build, with a command like: eas build --profile preview --platform ios --clear-cache
Once the build is done, install it on your device. Within your app, do whatever action will trigger the event call you set up.
If you go to the Firebase dashboard, you should see a count in the Users in last 30 minutes
section after a minute or two.
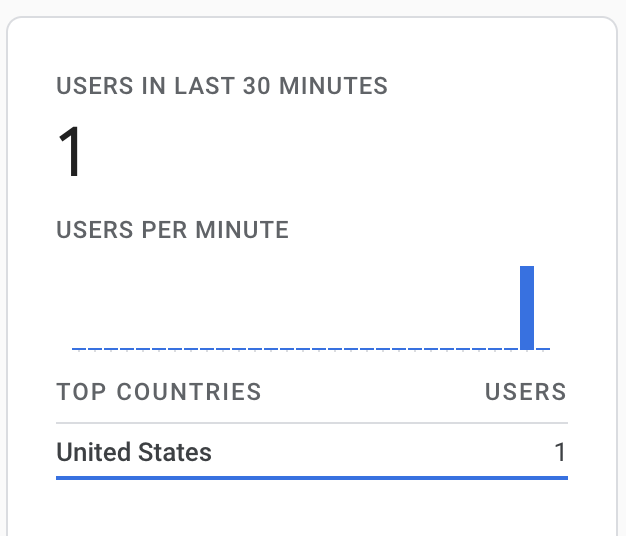
The events take up to 24 hours to show up in Firebase, so be patient.
References
Expo installation guide: https://docs.expo.dev/guides/using-firebase/#using-react-native-firebase
Firebase setup guide: https://rnfirebase.io/#managed-workflow
Firebase console: https://console.firebase.google.com/u/0/